What is enum ?
- The enum keyword is used to declare an enumeration
- A distinct type consisting of a set of named constants called the enumerator list
- Every enumeration type has an underlying type
- The default underlying type of the enumeration elements is int
- By default, the first enumerator has the value 0
- And the value of each successive enumerator is increased by 1
- EF 5 enum can have the Types as Byte, Int16, Int32, Int64 or SByte
Let's Take a Simple Example
E.g. 1 :
enum Days {Sat, Sun, Mon, Tue, Wed, Thu, Fri};
In this enumeration, Sat is 0, Sun is 1, Mon is 2, and so forth
E.g. 2 : Enumerators can have initializers to override the default values
enum Days {Sat=1, Sun, Mon, Tue, Wed, Thu, Fri};
In this enumeration, the sequence of elements is forced to start from 1 instead of 0
How to Use enum with Entity Framework 5 Code First ?
- This feature is Latest improvement of EF 5
Step 1 : Open Visual Studio 2012
Step 2 : File ==> New Project as EF5EnumSupport
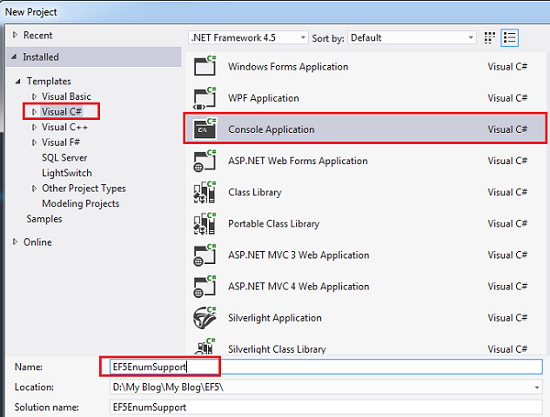
Step 3 : Right Click Your Solution and then Click Manage NuGet Packages...

Step 4 : Click Online Tab and Then Type Entity Framework 5 on Search Box
After that Select EntityFramework and Click Install

Step 5 : Click I Accept

After the above Step, below mentioned DLLs (Red Color) have added to the Project

Step 6 : Add ==> New Class and make it as enum Type Course
Course.cs

namespace EF5EnumSupport.Models
{
public enum Course
{
Mcsd = 1,
Mcse,
Mcsa,
Mcitp
}
}
Step 7 : Add ==> New Class as Student
Student.cs
namespace EF5EnumSupport.Models
{
public class Student
{
public int Id { get; set; }
public string Name { get; set; }
public Course Course { get; set; }
}
}
Step 8 : Create DbContext derived Class as StudentContext
StudentContext.cs
using System.Data.Entity;
namespace EF5EnumSupport.Models
{
public class StudentContext : DbContext
{
public DbSet<Student> Students { get; set; }
}
}
Step 9 : Console application's Main Method is as below
Program.cs
using System;
using System.Linq;
using EF5EnumSupport.Models;
namespace EF5EnumSupport
{
public class Program
{
static void Main(string[] args)
{
using (var context = new StudentContext())
{
context.Students.Add(new Student { Name = "Sampath Lokuge",
Course = Course.Mcsd });
context.SaveChanges();
var studentQuery = (from d in context.Students
where d.Course == Course.Mcsd
select d).FirstOrDefault();
if (studentQuery != null)
{
Console.WriteLine("Student Id: {0} Name: {1} Course : {2}",
studentQuery.Id,
studentQuery.Name,
studentQuery.Course);
}
}
}
}
}
The brand new enum support allows you to have enum properties in your entity classes

Step 10 : Run the Console Application
DEBUG ==> Start Without Debugging (Ctrl + F5)

Step 11 : Where's the Database ?
- By default the database will be created on the LocalDB instance
- The Entity Framework names the database after the fully qualified name of the derived context
- i.e. EF5EnumSupport.Models.StudentContextSQL Server Object Explorer in Visual Studio 2012 is as below
View Data on Students Table

What is LocalDb ?
- Microsoft SQL Server 2012 Express LocalDB is an execution mode of SQL Server Express targeted to program developers
- LocalDB installation copies a minimal set of files necessary to start the SQL Server Database Engine
That's It.You're Done.
Conclusion
- Entity Framework 5 brings a number of improvements and enum Support in Code First is one of them
- You can see that when you use enum type with your model class, Visual Studio 2012 gives intellisense support out of the box
- It's really awesome new feature of the Entity Framework 5
- Enjoy it
I hope this helps to You.Comments and feedback greatly appreciated.
If you feel it was a good article,Give me a +1.Thank You.
Happy Coding.
Another Article about Entity Framework 5
What is New in Entity Framework 5 ?
nice and very detailed, are you into trainings, whats ur objective?
ReplyDeleteHi Ganesh,
DeleteThanks for your feedback.
Actually My objective is learning latest things and share it with your'll.
Now tech blogging has become my hobby :)
Nice post. Always attach sample project.
ReplyDeleteHi askarmus,
DeleteThanks, I’m glad you enjoyed it!
Cool post, thank. I know that EF is growing.
ReplyDeleteSent from LinkedIn for iPad
-Mykhailo Basiuk-
Hi Mykhailo,
DeleteThanks for your feedback.
Keep in Touch.
Nice post and well explained... Thanks for sharing...
ReplyDeleteHi Safeer,
DeleteThanks,I’m glad you found it useful!
Thanks. It'is very good your explanation and examples.
ReplyDeleteCongratulations!
Hi Claudio,
DeleteYou're warmly welcome.
Stay in touch.
Good One Sampath!!!
ReplyDeletekeep posting more..
Hi Priyanka,
DeleteGlad to hear that it helped!
Keep in Touch.
Very good update. I am always wondering about why we can't use C# expressions in LINQ to SQL queries. Now Enum support is very useful one. Thanks to author.
ReplyDeleteHi Mukesh,
DeleteThanks, I’m glad you enjoyed it!
Keep in Touch.
If you modify the Student.cs file to have a collection of courses it does not work. Are enum collections not supported?
ReplyDeletepublic class Student
{
public Student()
{
this.Courses = new List();
}
public int Id { get; set; }
public string Name { get; set; }
public IList Courses { get; set; }
}
Hi Sammy,
DeleteYes.You're right.Entity Framework doesn't support having a collection of a Complex Type (such as enum) on an Entity type.
So,I don't know exactly what you're trying to achieve by using this (i.e. Business scenario).If you could put more details about your scenario then may be I could give more support.
its very useful . thks buddy.
ReplyDeleteHi Satish,
DeleteYou're warmly welcome.
Keep in Touch.
Great explanation thank you, i have a one query,which is How to add extra enums dynamically by using EF5 .
ReplyDelete