- It's a Tool to detect errors and potential problems in JavaScript code
- Can be used to enforce coding conventions
- Simple Tool that can very often spot your mistakes before you do
- It can save you from a lot of tedious debugging
- Lets you re-factor JavaScript code with greater confidence
- It's reduces the chances of you deploying broken code to your production server
What does JSHint do ?
- It identifies definite, objective errors in your code
- Such as mistyped keywords, mistyped variable names, mismatched parentheses, and other syntactical slip-ups
- It enforces some basic code style consistency rules
- Such as No trailing white space, no use of the == operator, etc.
- Depending on how you configure JSHint
How to Integrate JSHint with Visual Studio 2010 ?
Step 1. Open Visual Studio 2010
Step 2. Click Tools ===> Extension Manager
Step 3. Click "Online Gallery" Button
Step 4. Type "jslint" inside the Search box
Step 5. Click "Download" Button
Step 6. Click "Install" Button
Step 7. Click "Restart Now" Button for Restart Visual Studio 2010
That's It.You're Done.
How to Configure JSHint ?
Step 1. Click Tools ===> JS Lint Options... on Visual Studio 2010 menu
are as below
Step 3. Click "JSLint Options" and Then select
"JSHint" is as
below
How to Configure Global Variables of jQuery and Knockout ?
Step : Click "JSLint Options" and Then Type $ for jQuery and ko for Knockout inside the
"Predefined Vars" Text box with separator as Comma (,)
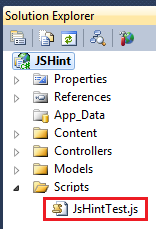
Let's do Small Demonstration
JsHintTest.js
function averageWeight(weights) {
var sum =
0, index;
for (index
= 0; index < weights.length; index += 1) {
sum += weights[index];
}
return sum
/ weights.length;
}
var myAverageWeight = averageWeight([1.75, 1.78, 1.82]);
After that, change the above function's Name as average and Parameter as values
JsHintTest.js file with Changes (with Errors)
function average(values) { // <-- This is the only line has been changed
function average(values) { // <-- This is the only line has been changed
var sum =
0, index;
for (index
= 0; index < weights.length; index += 1) {
sum += weights[index];
}
return sum
/ weights.length;
}
var myAverageWeight = averageWeight([1.75, 1.78, 1.82]);
- Then try to Save (Ctrl+S) the JsHintTest.js file
- After that It will show all the Errors are as below
You can see all the mistakes which you did by using JSHint very easily.
Would You Like to Test Your JS Code Online ?
Do You Need to Know More about JSHint ?
Conclusion
- JSHint is best when you’re writing fresh new code
- It needs a bit of configuration to give the best results
- JSHint is reliable and sophisticated Tool for find errors on JavaScript code files
- You could use JSHint for enforces some basic code style consistency rules
I hope this helps to You.Comments and feedback greatly appreciated.
If you feel it was a good article,Give me a +1.Thank You.
Happy Coding.
check this online js validator.
ReplyDeletehttp://www.jslint.com/
-Kanishka Panamaldeniya-
Hi Kanishka,
DeleteThanks for additional Information.
Keep in Touch.
Excellent post.
ReplyDeleteHi askarmus,
DeleteYou're warmly welcome.
Keep in Touch.
Hi sampath,
ReplyDeleteI tried that but there its not showing as error with the same demo given as creating a js file in script then renaming the function name also the parameter values. The java script error is not been shown but the four errors are listed as warnings.
-Vishnu Nadesh-
Hi Vishnu,
DeleteYes,You did “JSLint Options” setting mistake. So you have to set that is as "Warnnings --> Errors" as I showed in Step 2 under "How to Configure JSHint?".Then your problem should be vanished. Plz let me know If you need more help.
Hi Sampath,
ReplyDeleteThere's a small correction. You have mis-spelled JSLint as JSHint in couple of places.
I read most of your blogs and they are useful, thanks.
-Uchitha Chris. Ranasinghe-
Hi Uchitha,
DeleteThanks for your feedback.
Answer for Your Question :
Actually that is not a mistake.
Historically, JSHint is derived from the longer-established JSLint project from Douglas Crockford. The reason why JSHint exists is just to offer more flexibility: JSHint has a modest set of rules derived from common use, whereas JSLint is stricter and requires you to code exactly like the legendary Mr Crockford does.
If you need to configure for JSLint, then You can find this setting is shows as Step 3 Under "How to Configure JSHint ?" (just select JSLint from drop down box)
Sampath,
DeleteThanks man...I never knew about JSHint.
-Uchitha Chris. Ranasinghe-