- jQuery is a lightweight, "Write Less, Do More", JavaScript library.
- The purpose of jQuery is to make it much easier to use JavaScript on your website.
- jQuery takes a lot of common tasks that requires many lines of JavaScript code to accomplish, and wraps it into methods that you can call with a single line of code.
- jQuery also simplifies a lot of the complicated things from JavaScript, like AJAX calls and DOM manipulation.
- Document Object Model
- is the Backbone of every webpage
- web browser parses HTML and Javascript and maintains
a Data Model of what should be displayed to the user
The jQuery Library Contains the Following Features:
- HTML/DOM manipulation
- CSS manipulation
- HTML event methods
- Effects and animations
- AJAX
- Utilities
- jQuery has plugins for almost any task out there.
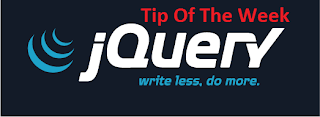
What is a jQuery Selector?
Selector Syntax
- A Selector identifies an HTML element tag that is used to manipulate with jQuery code.
- Selectors allow page elements to be selected
Selector Syntax
$(Selector Expression) OR jQuery(Selector Expression)
What are the Types of jQuery Selectors?
1. Class Selectors
$(".intro") - All elements with class="intro"
2. ID Selectors
$("#lastname") - The element with id=lastname
3. Element Selectors
$("p") - All p elements
How to Caching jQuery Selectors ?
What is Happening When We use Selector ?
- jQuery Traverses through all Elements of DOM every time
How Can Overcome this?
- You should Always Cache your Selections to some variable
Its Like this :
var myList = $('.myList');
myList.text("cached");
When to Use ?
- Using the Same Selection More than Once in your DOM Tree.
- e:g: Inside a jQuery Function Likes below
BAD Way
$(".myList").click( function() {
$(".myList").hide();
});
BEST Way
var myList = $(".myList");
myList.click( function() {
myList.hide(); // No need to re-select .myList since we cached it
});
More Tips for Optimizing jQuery Selector Performance :
1. Qualify your Selector strings when searching by class
- give Selector's Context as 2nd Parameter
- give Selector's Context as 2nd Parameter
- when the branches of your DOM tree become very deep
What is a Context ?
- jQuery has an optional second argument called context
- Its Limit the search within a specific node
- Great when you have a very Large DOM tree and need to find
e.g: All the <p> tags within a specific part of the DOM tree
- You can check to see what is the context of Selector by using context
Property.
e.g: $('p').context; //out put is => document
BAD Way
$(".your-class")
BEST Way
// get the node for the context
// get the node for the context
var context = $('#myContainer')[0];
$(".your-class", context )
2. Don't Qualify your selector when searching by Id.
- searching by Id uses the browsers native getElementById method (which is very fast)
BAD Way
$("div#your-id")
BEST Way
$("#your-id")
Conclusion
- Always Try to use Id of a Selector when its possible
- Always Do Caching for jQuery Selectors before it use
- Always Use Context with class Selectors
I hope this helps to You.Comments and feedback greatly appreciated.
Happy Coding.
jQuery Related Articles
How to Disable a Button Using Jquery and CSS ?
How to Enable jQuery Intellisense in VS 2010 ?
Great article, it was useful for me. thanks.
ReplyDelete- Aleksandr Golovatuy -
Hi Aleksandr,
DeleteThanks for your appreciation.
Do Keep in Touch.
Useful information, great, thanks author
ReplyDeleteHi MicrowebNET,
DeleteThanks for your response.
Do Keep in Touch.
Nice and useful basic....
ReplyDeleteVery helpful indeed.
Aref
Hi devintonet,
DeleteThanks for your reply.
Do Keep in Touch.
Hi Sampath,
ReplyDeleteGood article. lot of information with best practises.
Regards
Sanjaya Maduranga De Silva
Hi Maduranga,
DeleteThanks.Your most welcome.
Malli Ela kollekne dan
ReplyDeleteHi Padmathilaka,
DeleteThanks for your reply.
Do Keep in Touch.
keep in touch
ReplyDeleteHi,
DeleteThanks for your feedback.
Do Keep in Touch.
Great article! This will definitely come in handy.
ReplyDeleteHi Terence,
DeleteThanks for your appreciation.
Do Keep in Touch.
likes this.
ReplyDelete-Todd McFarlane-Smith-
Hi Todd,
DeleteNice to here you.Do Keep in Touch.
Great article.
ReplyDelete-Terence Hall-
Hi Terence,
DeleteThanks.Your welcome.
Do Keep in Touch.
Hi,
ReplyDeleteNice article. Thanks for sharing.
One question though. I'm not clear about the difference between local vs online. Whether it's local or online, JavaScript execution happens inside the browser, and it should make no difference (apart from script download times). Once the scripts and page is loaded, it should be same for both local and online, if the document is same. Isn't it?
Regards,
Yohan.
Hi Yohan,
DeleteThanks for your feedback.
About Your Question:
Local Means - Tested on local machine with native code and also "Debug" mode.And without host on IIS. That means under development server.
Online Means - Tested on IIS which was on remote server and "Release" mode.
Yes your point is right.For online scenario I didn't remove script download time.But when you compare single stream (either Online or local) then you can have rough idea about differences.Comparison between online vs Local is not suitable because the way I got the time.
Thanks.Do Keep in Touch.
I think this code
ReplyDelete$(".myList").click( function() {
$(".myList").hide();
});
can be replaced by:
$(".myList").click( function() {
$(this).hide();
});
-Roger Manich-
Hi Roger,
DeleteYes you can write that way.But my concern is how to cache the selector.When you write that way still it traverse DOM tree to find the myList class.When function is in inside the for loop its really costly.
I hope you get my point.Good luck.
like this.
ReplyDelete-Henrique Adriano-
Hi Henrique,
DeleteThanks for your response.
Do Keep in Touch.
nice article.........
ReplyDelete-SANJAY YADAV-
Hi Sanjay,
DeleteThanks for your feedback.
Do keep in touch.
Very good overview on jQuery selector performance. Simple and to the point :) keep it up.
ReplyDeleteHi Mark,
DeleteThanks for your appreciation.
Do Keep in Touch.
Generally search by #Id is faster as compare to class selector.
ReplyDelete$('tag.class') is faster than just $('.class').
Next always specify the scope in selector (this).
-Adnan Samuel-
e.g. If we have element reference and we are using class specifier then we can specify as $(".classname", element) and obviously it is faster than just $('.class'). See jquery documentation for detail. :)
Delete-Adnan Samuel-
Hi Adnan,
DeleteThanks for your additional information.
Do keep in touch.
Thnx it really helped !
ReplyDeleteHi Ninad,
DeleteYour welcome.
Do Keep in Touch.
This is a fantastic article.
ReplyDeleteHi DP,
DeleteThanks for your appreciation.
Do Keep in Touch.
Really good article. I am very new to JQuery but got all your points.
ReplyDeleteThanks! Keep writing :)
Hi කල්,
DeleteNice to hear you.If you having any problem let me know.I will give any support for you.
Thanks for your feedback
Do Keep in Touch.
likes this.
ReplyDelete-Mukesh Selvaraj-
Hi Mukesh,
DeleteThanks for your feedback.
Do Keep in Touch.
Hi Sampath,
ReplyDeleteGreat work buddy! :).
Keep it going on
Best Regards,
Nandun
Hi Nandun,
DeleteYour most welcome.
Do Keep in Touch.
Hello Sampath!
ReplyDeleteI want to say THANK YOU for giving me some important information to grow my knowledge OK.
Thanking you.
Bye Sampath.
-Muhammed Nuzli-
Hi Muhammed,
DeleteThanks for your appreciation.
Do Keep in Touch.
Hi,
ReplyDeleteThank you very much.
-Dasun Tharaka De Silva-
Hi Dasun,
DeleteYour welcome.
Do Keep in Touch.
Great article on performance improvement. I'll use this technique on a prototyping project this week.
ReplyDeleteThanks!
Hi Jim,
DeleteNice to hear you.Good luck for your new project.If you need any help about jQuery please let me know.
Do Keep in Touch.